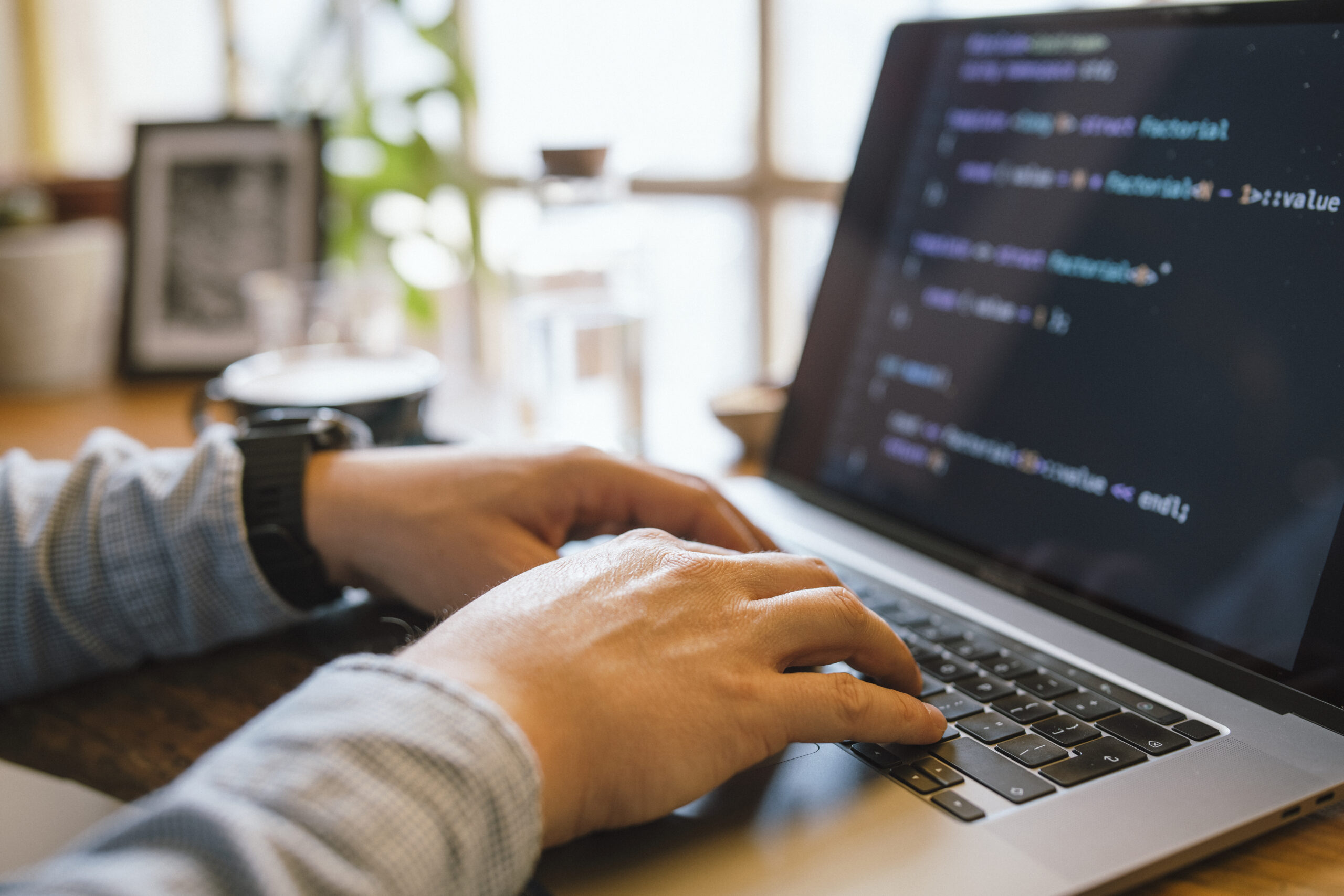
Debugging is Among the most critical — however typically forgotten — competencies in a developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and Mastering to Assume methodically to unravel complications competently. Whether or not you're a beginner or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of aggravation and significantly boost your productivity. Listed below are numerous techniques to aid developers amount up their debugging activity by me, Gustavo Woltmann.
Learn Your Equipment
One of many quickest ways builders can elevate their debugging capabilities is by mastering the equipment they use each day. Whilst writing code is a person Component of advancement, understanding how to connect with it proficiently through execution is equally essential. Fashionable progress environments arrive equipped with highly effective debugging capabilities — but many builders only scratch the surface area of what these applications can do.
Take, one example is, an Built-in Advancement Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment let you set breakpoints, inspect the worth of variables at runtime, stage through code line by line, and in many cases modify code within the fly. When used the right way, they Permit you to notice just how your code behaves in the course of execution, which is priceless for tracking down elusive bugs.
Browser developer equipment, like Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, watch network requests, watch genuine-time performance metrics, and debug JavaScript inside the browser. Mastering the console, sources, and community tabs can change disheartening UI troubles into manageable jobs.
For backend or method-level builders, equipment like GDB (GNU Debugger), Valgrind, or LLDB provide deep Command in excess of working processes and memory administration. Learning these resources may have a steeper Mastering curve but pays off when debugging efficiency issues, memory leaks, or segmentation faults.
Outside of your IDE or debugger, turn out to be relaxed with version Command programs like Git to know code history, obtain the exact minute bugs were launched, and isolate problematic variations.
In the end, mastering your instruments usually means likely beyond default settings and shortcuts — it’s about establishing an personal knowledge of your advancement natural environment so that when problems crop up, you’re not shed at nighttime. The greater you understand your instruments, the greater time you'll be able to shell out solving the actual difficulty rather than fumbling via the procedure.
Reproduce the trouble
One of the most crucial — and often missed — ways in productive debugging is reproducing the trouble. Just before leaping to the code or building guesses, builders will need to produce a reliable natural environment or circumstance where by the bug reliably appears. Without having reproducibility, repairing a bug turns into a sport of opportunity, usually leading to squandered time and fragile code improvements.
Step one in reproducing a challenge is collecting as much context as possible. Ask questions like: What steps resulted in the issue? Which ecosystem was it in — progress, staging, or creation? Are there any logs, screenshots, or error messages? The greater element you may have, the less difficult it becomes to isolate the precise circumstances underneath which the bug occurs.
As you’ve collected more than enough data, attempt to recreate the situation in your local natural environment. This could signify inputting exactly the same facts, simulating comparable person interactions, or mimicking system states. If The problem seems intermittently, think about producing automatic exams that replicate the sting conditions or state transitions included. These checks not merely help expose the situation but in addition prevent regressions Down the road.
Occasionally, The problem may very well be surroundings-specific — it might come about only on particular running systems, browsers, or beneath unique configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these bugs.
Reproducing the issue isn’t merely a action — it’s a mindset. It needs persistence, observation, and also a methodical solution. But when you can continually recreate the bug, you might be already halfway to fixing it. Using a reproducible circumstance, You may use your debugging applications more successfully, examination likely fixes safely and securely, and converse extra Evidently with your crew or people. It turns an summary criticism right into a concrete challenge — and that’s in which developers thrive.
Study and Realize the Error Messages
Error messages will often be the most beneficial clues a developer has when some thing goes Improper. As an alternative to observing them as annoying interruptions, developers must find out to treat mistake messages as immediate communications with the technique. They typically let you know precisely what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start out by looking through the message carefully As well as in whole. Several developers, specially when beneath time stress, look at the primary line and right away start building assumptions. But deeper in the mistake stack or logs could lie the accurate root cause. Don’t just duplicate and paste mistake messages into search engines like google and yahoo — read through and comprehend them to start with.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or simply a logic error? Will it level to a selected file and line amount? What module or functionality induced it? These issues can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to be aware of the terminology with the programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Studying to acknowledge these can dramatically increase your debugging procedure.
Some glitches are imprecise or generic, and in Individuals scenarios, it’s essential to examine the context where the mistake occurred. Check out similar log entries, input values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings both. These typically precede larger sized problems and provide hints about probable bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them correctly turns chaos into clarity, assisting you pinpoint troubles speedier, cut down debugging time, and turn into a more effective and assured developer.
Use Logging Properly
Logging is The most highly effective applications inside of a developer’s debugging toolkit. When used effectively, it provides real-time insights into how an application behaves, helping you understand what’s happening under the hood without having to pause execution or move in the code line by line.
A good logging strategy starts off with recognizing what to log and at what amount. Prevalent logging degrees include things like DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for detailed diagnostic data through development, Information for standard events (like profitable start out-ups), Alert for opportunity difficulties that don’t split the appliance, ERROR for actual issues, and Lethal if the program can’t carry on.
Avoid flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure essential messages and decelerate your technique. Give attention to essential occasions, point out alterations, input/output values, and important selection points as part of your code.
Format your log messages Evidently and consistently. Include things like context, including timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Enable you to track how variables evolve, what ailments are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re In particular beneficial in generation environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. By using a perfectly-believed-out logging tactic, you can decrease the time it will require to identify problems, get further visibility into your apps, and Increase the General maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly establish and fix bugs, developers need to technique the procedure similar to a detective resolving a mystery. This state of mind aids break down intricate challenges into workable parts and adhere to clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the indications of the problem: error messages, incorrect output, or overall performance problems. Much like a detective surveys a crime scene, gather as much applicable info as you'll be able to with no leaping to conclusions. Use logs, examination situations, and consumer studies to piece with each other a clear picture of what’s happening.
Subsequent, type hypotheses. Request oneself: What could possibly be leading to this conduct? Have any adjustments just lately been manufactured on the codebase? Has this situation transpired prior to under similar circumstances? The goal should be to slender down options and discover possible culprits.
Then, check your theories systematically. Make an effort to recreate the challenge in the controlled surroundings. If you suspect a selected functionality or part, isolate it and confirm if The problem persists. Like a detective conducting interviews, ask your code issues and Allow the results guide you nearer to the truth.
Pay out close attention to small particulars. Bugs normally disguise while in the least envisioned destinations—just like a lacking semicolon, an off-by-one particular error, or maybe a race affliction. Be comprehensive and affected individual, resisting the urge to patch The problem without entirely comprehending it. Momentary fixes could disguise the true problem, only for it to resurface afterwards.
Finally, retain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging method can help you save time for potential difficulties and assist Other folks understand your reasoning.
By pondering just like a detective, builders can sharpen their analytical abilities, tactic problems methodically, and turn into more practical at uncovering hidden concerns in advanced systems.
Publish Assessments
Crafting tests is one of the best strategies to help your debugging skills and All round growth performance. Tests not just support capture bugs early and also function a safety net that provides you assurance when producing adjustments to the codebase. A very well-tested application is easier to debug because it enables you to pinpoint precisely exactly where and when an issue occurs.
Start with unit tests, which focus on individual functions or modules. These small, isolated tests can quickly expose whether or not a specific bit of logic is Doing the job as predicted. Every time a examination fails, you quickly know the place to search, drastically minimizing time invested debugging. Device assessments are Specially valuable for catching regression bugs—troubles that reappear right after Formerly get more info becoming preset.
Upcoming, integrate integration tests and close-to-conclusion exams into your workflow. These assist ensure that many areas of your application get the job done collectively smoothly. They’re significantly valuable for catching bugs that happen in elaborate programs with numerous factors or companies interacting. If some thing breaks, your checks can let you know which Element of the pipeline failed and under what problems.
Creating assessments also forces you to think critically about your code. To check a characteristic properly, you require to know its inputs, envisioned outputs, and edge situations. This level of knowledge By natural means potential customers to better code framework and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a powerful initial step. When the test fails persistently, you could give attention to repairing the bug and watch your check move when The difficulty is resolved. This strategy makes certain that precisely the same bug doesn’t return Down the road.
In short, composing assessments turns debugging from the frustrating guessing recreation right into a structured and predictable course of action—helping you catch a lot more bugs, speedier plus more reliably.
Take Breaks
When debugging a tricky situation, it’s uncomplicated to become immersed in the trouble—observing your monitor for several hours, seeking solution following Remedy. But The most underrated debugging instruments is actually stepping absent. Getting breaks allows you reset your intellect, reduce frustration, and often see the issue from the new standpoint.
If you're far too near the code for far too very long, cognitive exhaustion sets in. You would possibly start out overlooking evident glitches or misreading code that you just wrote just hrs previously. Within this state, your Mind results in being fewer successful at challenge-fixing. A brief stroll, a coffee break, or simply switching to another undertaking for 10–15 minutes can refresh your focus. Lots of builders report locating the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid prevent burnout, Primarily through more time debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but will also draining. Stepping absent enables you to return with renewed Electrical power and also a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you before.
When you’re stuck, a fantastic rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment break. Use that point to move all around, stretch, or do anything unrelated to code. It may come to feel counterintuitive, especially underneath tight deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks just isn't an indication of weakness—it’s a wise tactic. It gives your brain Place to breathe, increases your viewpoint, and can help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is an element of resolving it.
Discover From Every single Bug
Every bug you experience is much more than simply A short lived setback—it's an opportunity to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can teach you some thing useful when you go to the trouble to replicate and analyze what went Incorrect.
Commence by inquiring on your own a handful of vital questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it are caught before with improved tactics like device tests, code opinions, or logging? The responses generally expose blind places with your workflow or comprehension and help you build stronger coding patterns going ahead.
Documenting bugs can even be an outstanding practice. Hold a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and what you acquired. Eventually, you’ll begin to see designs—recurring problems or typical mistakes—you could proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with all your peers may be especially impressive. No matter whether it’s by way of a Slack message, a brief create-up, or A fast expertise-sharing session, aiding others steer clear of the identical issue boosts staff efficiency and cultivates a much better Mastering tradition.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll get started appreciating them as vital elements of your progress journey. In any case, a lot of the ideal builders usually are not those who create fantastic code, but people who consistently discover from their faults.
In the end, Just about every bug you repair adds a completely new layer in your talent established. So up coming time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more capable developer as a result of it.
Summary
Improving your debugging expertise usually takes time, apply, and persistence — although the payoff is huge. It helps make you a far more economical, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be superior at what you do.